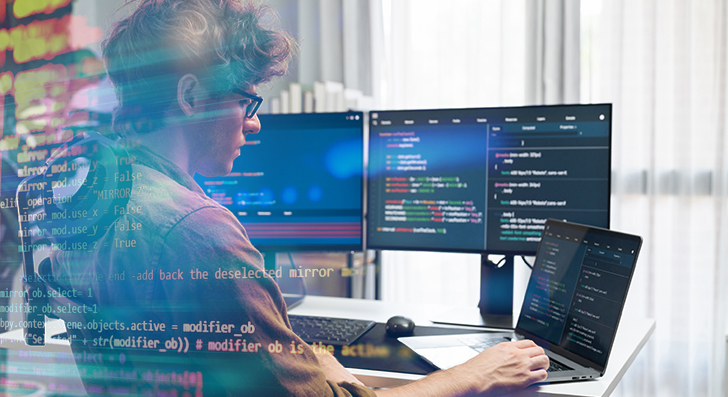
Scalability suggests your software can tackle expansion—far more users, extra facts, plus much more website traffic—devoid of breaking. Like a developer, building with scalability in your mind saves time and worry later on. Below’s a transparent and realistic guideline to assist you to start off by Gustavo Woltmann.
Style for Scalability from the beginning
Scalability is not some thing you bolt on afterwards—it should be section of your approach from the start. Numerous apps fail when they mature quickly for the reason that the initial structure can’t cope with the extra load. Being a developer, you need to Consider early regarding how your program will behave stressed.
Begin by designing your architecture to get adaptable. Stay away from monolithic codebases wherever every little thing is tightly related. Rather, use modular style and design or microservices. These patterns break your application into smaller sized, impartial sections. Every module or provider can scale By itself without affecting The full method.
Also, think of your databases from working day 1. Will it need to have to take care of a million customers or maybe 100? Choose the appropriate form—relational or NoSQL—based on how your information will expand. Prepare for sharding, indexing, and backups early, Even when you don’t have to have them however.
Yet another vital stage is to prevent hardcoding assumptions. Don’t compose code that only performs underneath latest disorders. Give thought to what would happen if your consumer base doubled tomorrow. Would your app crash? Would the database slow down?
Use style patterns that support scaling, like message queues or event-pushed methods. These assist your app handle more requests without having overloaded.
After you Establish with scalability in your mind, you're not just getting ready for achievement—you are decreasing future problems. A perfectly-prepared program is easier to maintain, adapt, and improve. It’s better to arrange early than to rebuild later on.
Use the correct Databases
Picking out the appropriate database is a critical Section of creating scalable programs. Not all databases are built a similar, and utilizing the Incorrect you can sluggish you down or perhaps induce failures as your app grows.
Begin by being familiar with your data. Could it be extremely structured, like rows inside of a desk? If yes, a relational databases like PostgreSQL or MySQL is an effective in good shape. These are typically powerful with relationships, transactions, and regularity. They also guidance scaling strategies like read replicas, indexing, and partitioning to manage more website traffic and information.
If the info is more adaptable—like user exercise logs, merchandise catalogs, or documents—look at a NoSQL choice like MongoDB, Cassandra, or DynamoDB. NoSQL databases are superior at handling massive volumes of unstructured or semi-structured data and will scale horizontally much more quickly.
Also, consider your read through and generate patterns. Will you be doing a lot of reads with much less writes? Use caching and skim replicas. Have you been handling a large produce load? Look into databases that could tackle higher publish throughput, or simply event-primarily based knowledge storage methods like Apache Kafka (for short term facts streams).
It’s also good to Believe ahead. You may not need to have State-of-the-art scaling options now, but choosing a database that supports them indicates you gained’t will need to modify afterwards.
Use indexing to hurry up queries. Stay clear of unnecessary joins. Normalize or denormalize your information according to your accessibility designs. And often check databases general performance when you mature.
To put it briefly, the right databases depends on your application’s composition, velocity demands, And just how you hope it to mature. Choose time to select correctly—it’ll preserve plenty of problems later.
Improve Code and Queries
Speedy code is essential to scalability. As your app grows, just about every modest delay adds up. Improperly penned code or unoptimized queries can decelerate efficiency and overload your program. That’s why it’s crucial to build economical logic from the beginning.
Commence by composing thoroughly clean, simple code. Stay clear of repeating logic and remove something unnecessary. Don’t pick the most sophisticated Answer if a straightforward one particular functions. Keep your capabilities limited, focused, and straightforward to test. Use profiling applications to seek out bottlenecks—locations where by your code normally takes as well extensive to run or employs an excessive amount of memory.
Future, have a look at your databases queries. These typically gradual factors down more than the code by itself. Make sure Just about every query only asks for the information you really have to have. Stay away from Find *, which fetches every little thing, and instead decide on unique fields. Use indexes to speed up lookups. And prevent performing a lot of joins, Primarily across substantial tables.
Should you see exactly the same facts being requested time and again, use caching. Store the outcomes briefly applying resources like Redis or Memcached and that means you don’t really have to repeat costly functions.
Also, batch your databases operations whenever you can. As an alternative to updating a row one after the other, update them in teams. This cuts down on overhead and makes your app far more successful.
Make sure to test with big datasets. Code and queries that perform great with 100 records may well crash whenever they have to manage one million.
To put it briefly, scalable applications are fast apps. Keep your code tight, your queries lean, and use caching when needed. These measures help your application stay smooth and responsive, even as the load increases.
Leverage Load Balancing and Caching
As your app grows, it has to handle more users and much more visitors. If every thing goes by means of a single server, it can promptly turn into a bottleneck. That’s the place load balancing and caching can be found in. These two resources assist keep your application rapid, steady, and scalable.
Load balancing spreads incoming targeted traffic across numerous servers. Rather than a single server carrying out all of the work, the load balancer routes users to distinctive servers dependant on availability. What this means is no solitary server gets overloaded. If a person server goes down, the load balancer can send out traffic to the Other people. Applications like Nginx, HAProxy, or cloud-dependent solutions from AWS and Google Cloud make this very easy to create.
Caching is about storing information quickly so it could be reused rapidly. When buyers ask for exactly the same information yet again—like a product web site or maybe a profile—you don’t must fetch it from the databases whenever. You are able to provide it from your cache.
There's two widespread kinds of caching:
one. Server-side caching (like Redis or Memcached) outlets information in memory for speedy accessibility.
two. Client-facet caching (like browser caching or CDN caching) merchants static files near to the person.
Caching decreases databases load, improves pace, and makes your application more productive.
Use caching for things which don’t modify normally. And usually ensure that your cache is up-to-date when details does modify.
To put it briefly, load balancing and caching are easy but highly effective tools. Collectively, they assist your app manage additional users, remain rapid, and recover from difficulties. If you intend to develop, you may need both of those.
Use Cloud and Container Resources
To create scalable apps, you need resources that allow your application improve easily. That’s in which cloud platforms and containers can be found in. They provide you adaptability, reduce setup time, and make scaling A lot smoother.
Cloud platforms like Amazon Website Services (AWS), Google Cloud Platform (GCP), and Microsoft Azure let you rent servers and providers as you may need them. You don’t should invest in components or guess future capacity. When visitors raises, you'll be able to incorporate far more assets with just a couple clicks or quickly using vehicle-scaling. When traffic drops, you can scale down to economize.
These platforms also give products and services like managed databases, storage, load balancing, and stability applications. You could deal with making your application as opposed to handling infrastructure.
Containers are Yet another important tool. A container offers your application and all the things it ought to run—code, libraries, settings—into one device. This causes it to be straightforward to move your application amongst environments, out of your laptop to your cloud, with no surprises. Docker is the most well-liked tool for this.
Once your app utilizes numerous containers, tools like Kubernetes make it easier to deal with them. Kubernetes handles deployment, scaling, and recovery. If a person portion of one's application crashes, it restarts it instantly.
Containers also allow it to be straightforward to independent parts of your application into solutions. You could update or scale elements independently, which is perfect for overall performance and reliability.
Briefly, utilizing cloud and container applications implies you could scale quickly, get more info deploy easily, and Recuperate immediately when troubles happen. If you need your application to expand without the need of limitations, start out using these equipment early. They help you save time, minimize possibility, and allow you to continue to be focused on constructing, not correcting.
Keep track of Almost everything
For those who don’t check your software, you received’t know when things go Improper. Checking allows you see how your app is doing, location challenges early, and make much better selections as your application grows. It’s a vital Portion of making scalable units.
Begin by tracking simple metrics like CPU utilization, memory, disk Place, and reaction time. These show you how your servers and solutions are carrying out. Equipment like Prometheus, Grafana, Datadog, or New Relic may help you obtain and visualize this details.
Don’t just monitor your servers—keep track of your app also. Keep watch over just how long it requires for end users to load web pages, how frequently faults happen, and where they occur. Logging tools like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly will help you see what’s happening inside your code.
Set up alerts for important problems. For example, if your reaction time goes higher than a Restrict or maybe a provider goes down, you must get notified quickly. This aids you resolve problems quick, often prior to users even notice.
Checking can be beneficial whenever you make changes. For those who deploy a different attribute and see a spike in faults or slowdowns, you may roll it back again prior to it results in authentic injury.
As your app grows, website traffic and info improve. Without the need of monitoring, you’ll miss indications of problems until it’s too late. But with the appropriate resources set up, you remain on top of things.
In a nutshell, checking will help you keep your application reliable and scalable. It’s not almost spotting failures—it’s about knowledge your method and ensuring that it works very well, even under pressure.
Closing Thoughts
Scalability isn’t only for significant firms. Even small apps have to have a powerful Basis. By designing diligently, optimizing properly, and utilizing the right equipment, you can Create applications that develop efficiently without breaking under pressure. Start out small, Feel significant, and Develop sensible.